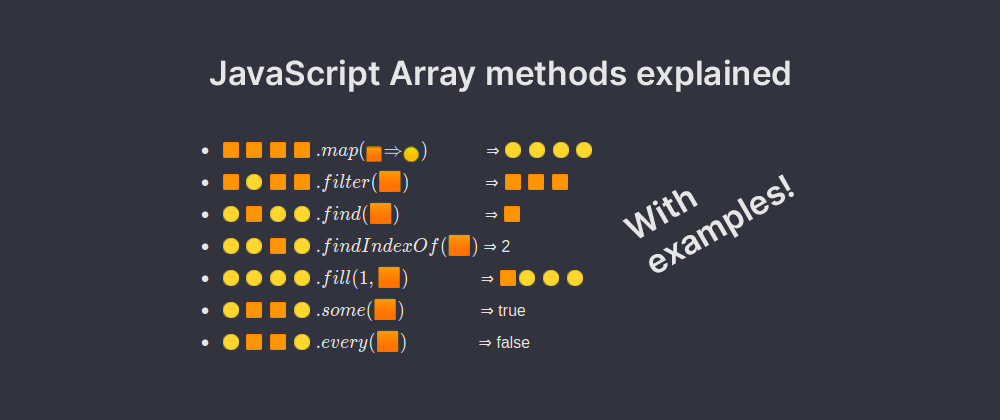
JavaScript Array methods explained with examples
7 min read
Yes, this is yet another article about JavaScript Array methods. There are plenty of great articles about the subject around the internet, so, why did I write this one?
After interacting with multiple of my mentees over the years, either interns or junior developers, I realized most of them need a tutorial to understand JavaScript Array methods. I decided to write this article to have an example at hand that I can use to teach and guide them, and to provide a quick introduction to the most important methods. Also, this helps me to refresh my memory about some I use the less.
If you are a beginner at JavaScript or Node, I hope you'll find it very helpful. But even to experienced developers, this article may come in handy to help you brush up on things as you need. So let's start!
First, a few considerations about arrays in JavaScript
Arrays in JavaScript are not like in other languages, such as Java. They also start at index: 0
, but have some differences:
In JavaScript, arrays can be a collection of elements of any type. This means that you can create an array with elements of type String, Boolean, Number, Objects, and even other Arrays. That's not the case in TypeScript, where arrays can only contain elements of the same type.
// javScript Array
const jsArray = [1234, true, 'hello', { subject: 'world' }]
// TypeScript Array of strings
const tsArray: string[] = ['hello', 'world']
Also, JavaScript arrays are not of fixed length. You can add or remove elements to/from them at anytime, effectively updating their length
.
const array = ['🍎', '🍉', '🍒']
console.log(array.length) // 3
array.push('🍌')
console.log(array.length) // 4
Creating an array
We can create an array in several ways, bust the most common ones are:
// Just assigning an array with their values to a variable
const array1 = ['🍎', '🍉', '🍒', '🍌', '🥝']
// Using Array constructor
const array2 = new Array('🍎', '🍉', '🍒', '🍌', '🥝')
All the above creates the same array in memory. Other useful ways are Array.of() or Array.from() methods.
How to add and remove elements to/from an array
We can use the method push() to add elements to the end of an array. If we want to add elements to the beginning of the array we must use the unshift() method instead. To add elements to the middle (or a custom index) of the array we can use the splice() method.
const array = ['🍎', '🍉', '🍒', '🍌', '🥝']
array.push('🥑') // ["🍎", "🍉", "🍒", "🍌", "🥝", "🥑"]
array.unshift('🍋') // ["🍋", "🍎", "🍉", "🍒", "🍌", "🥝", "🥑"]
// Add at index 2 a total of 1 element with value '🍑'
array.splice(2, 1, '🍑') // ["🍋", "🍎", "🍑", "🍉", "🍒", "🍌", "🥝", "🥑"]
To remove elements we can use the pop() and shift() methods, that works the oposite that previous ones. But also, we can use the splice() method to remove elements from the middle of the array.
const array = ['🍎', '🍉', '🍒', '🍌', '🥝', '🥑']
const avocado = array.pop() // avocado = '🥑'; array = ["🍎", "🍉", "🍒", "🍌", "🥝"]
const apple = array.shift() // apple = '🍎'; array = ["🍉", "🍒", "🍌", "🥝"]
// Remove starting from index 1 a total of 2 elements
const middle = array.splice(1, 2) // middle = ["🍒", "🍌"]; array = ["🍉", "🥝"]
How to clone an array
To make a copy of an array we can use the slice() method or the spread (...) operator:
const array = ['🍉', '🍎', '🍒']
const copy1 = array.slice() // copy1 = ["🍉", "🍎", "🍒"]
copy1.pop() // copy1 = ["🍉", "🍎"]; array = ["🍉", "🍎", "🍒"]
const copy2 = [...array] // copy2 = ["🍉", "🍎", "🍒"]
copy2.shift() // copy2 = ["🍎", "🍒"]; array = ["🍉", "🍎", "🍒"]
Array destructuring
We can get the values inside an array directly to variables using destructuring, like this:
const fruits = ['🍉', '🍎', '🍒']
const [first, second, third] = fruits // first = "🍉"; second = "🍎"; third = "🍒"
const [first, , third] = fruits // first = "🍉"; third = "🍒"
const [first, ...rest] = fruits // first = "🍉"; rest = ["🍎", "🍒"]
In the above lines, array content won't changed. Just new variables containing the value/s destructured will be created without modifying the original array.
Useful methods for arrays
Now, let's see some useful methods for arrays that are commonly used in JavaScript ecosystem.
concat()
The concat() method is used to merge two or more arrays. This method does not change the existing arrays, but instead returns a new array.
const fruits = ['🍉', '🍎', '🍒', '🍌']
const vegetables = ['🧅', '🌽', '🥕', '🥦']
const food = fruits.concat(vegetables)
// ["🍉", "🍎", "🍒", "🍌", "🧅", "🌽", "🥕", "🥦"]
join()
The join() method creates and returns a new string by concatenating all of the elements in an array.
const vegetables = ['🧅', '🌽', '🥕', '🥦']
const vegetablesGroup = vegetables.join('') // "🧅🌽🥕🥦"
const vegetablesDashed = vegetables.join('-') // "🧅-🌽-🥕-🥦"
some()
The some() method tests whether at least one element in the array passes the test implemented by the provided function. It returns true
if, in the array, it finds an element for which the provided function returns true; otherwise it returns false
. It doesn't modify the array.
const fruits = ['🍉', '🍎', '🍒', '🍌']
const bananaExists = fruits.some(fruit => fruit === '🍌')) // true
const cornExists = fruits.some(fruit => fruit === '🌽')) // false
every()
The every() method tests whether all elements in the array pass the test implemented by the provided function. It returns a Boolean value.
const fruits = ['🍉', '🍎', '🍒', '🍌']
const bananas = ['🍌', '🍌', '🍌']
const allBananas1 = fruits.every((fruit) => fruit === '🍌') // false
const allBananas2 = bananas.every((fruit) => fruit === '🍌') // true
fill()
The fill() method changes all elements in an array to a static value, from a start index (default 0
) to an end index (default array.length
). It returns the modified array.
const fruits = ['🍉', '🍎', '🍒', '🍌']
const lemons = fruits.fill('🍋') // ["🍋", "🍋", "🍋", "🍋"]
// Fill from index 1 (included) to index 3 (excluded)
const lemonsAndApples = fruits.fill('🍎', 1, 3) // ["🍋", "🍎", "🍎", "🍋"]
find()
The find() method returns the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined
is returned.
const fruits = ['🍉', '🍎', '🍒', '🍌']
const cherry = fruits.find((fruit) => fruit === '🍒') // "🍒"
const lemon = fruits.find((fruit) => fruit === '🍋') // undefined
findIndex()
The findIndex() method method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1
, indicating that no element passed the test.
const fruits = ['🍉', '🍎', '🍒', '🍌']
const cherryIndex = fruits.findIndex((fruit) => fruit === '🍒') // 2
const lemonIndex = fruits.findIndex((fruit) => fruit === '🍋') // -1
filter()
The filter() method creates a new array with all elements that pass the test implemented by the provided function. It does not change the original array.
const fruits = ['🍉', '🍎', '🍌', '🍌']
const onlyBananas = fruits.filter((fruit) => fruit === '🍌') // ["🍌", "🍌"]
const onlyLemons = fruits.filter((fruit) => fruit === '🍋') // []
includes()
The includes() method determines whether an array includes a certain value among its entries, returning true
or false
as appropriate.
const vegetables = ['🧅', '🌽', '🥕', '🥦']
const includesCorn = vegetables.includes('🌽') // true
const includesTomato = vegetables.includes('🍅') // false
forEach()
The forEach() method executes a provided function once for each array element.
const vegetables = ['🧅', '🌽', '🥕', '🥦']
vegetables.forEach((vegetable) => console.log(vegetable))
// "🧅"
// "🌽"
// "🥕"
// "🥦"
map()
The map() method creates a new array populated with the results of calling a provided function on every element in the calling array. It does not change the original array.
const vegetables = ['🧅', '🌽', '🥕', '🥦']
const doubledVegetables = vegetables.map((vegetable) => vegetable + vegetable)
// ["🧅🧅", "🌽🌽", "🥕🥕", "🥦🥦"]
sort()
The sort() method sorts the elements of an array in place and returns the sorted array. The default sort order is ascending, built upon converting the elements into strings, then comparing their sequences of UTF-16 code units values.
const fruits = ['🍉', '🍎', '🍒', '🍌', '🍉', '🍒', '🍎', '🍌']
fruits.sort() // ["🍉", "🍉", "🍌", "🍌", "🍎", "🍎", "🍒", "🍒"]
reverse()
The reverse() method reverses an array in place. The first array element becomes the last, and the last array element becomes the first.
const fruits = ['🍉', '🍎', '🍒', '🍌']
fruits.reverse() // ["🍌", "🍒", "🍎", "🍉"]
That's it!
I hope you've found this article insightful, and that it helps you understand JavaScript arrays more clearly. I have other articles about node and JS/TS that might be useful for you too. You can check my node articles or, if you don't know where to start, might How to convert callbacks into promises can be a good next lecture.
See you!